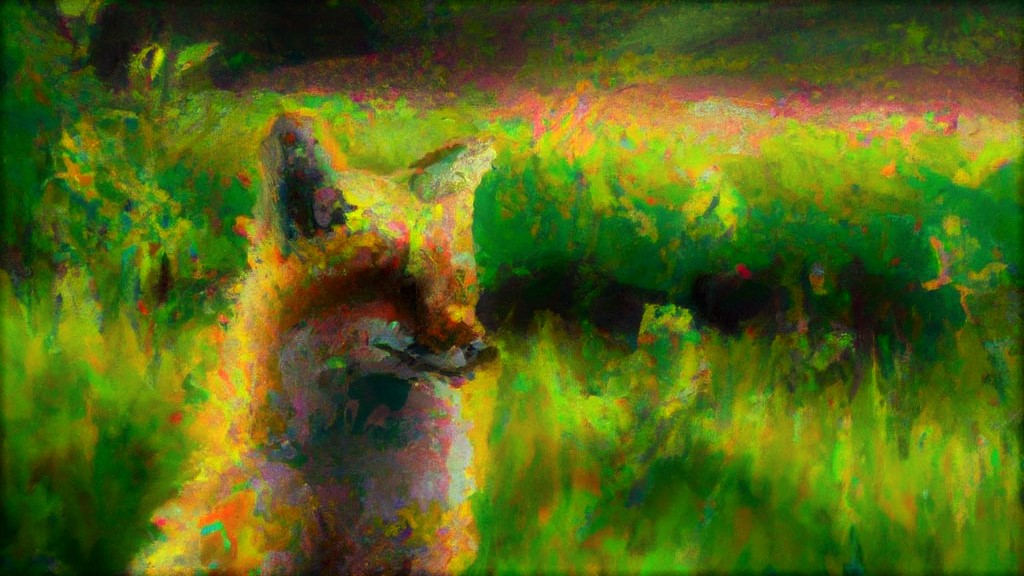
How to Change a Switch Statement to an Object in JavaScript
In JavaScript, you can substitute a switch statement with an object.
The function below uses a switch statement:
function whoPlaysWhere(val) {
let answer = "";
switch (val) {
case "Steph Curry":
answer = "Golden State Warriors";
break;
case "LeBron James":
answer = "Los Angeles Lakers";
break;
case "Kevin Durant":
answer = "Brooklyn Nets"
break;
case "Giannis Antetokounmpo" :
answer = "Milwaukee Bucks"
break;
}
return answer;
}
The function below shows an object that achieves the same results:
function whoPlaysWhere(val) {
let answer = "";
const players = {
"Steph Curry" : "Golden State Warriors",
"LeBron James" : "Los Angeles Lakers",
"Kevin Durant" : "Brooklyn Nets",
"Giannis Antetokounmpo" : "Milwaukee Bucks",
}
answer = players[val];
return answer;
}
The function below will return the same results as the one above:
function whoPlaysWhereObject(val) {
return {
"Steph Curry" : "Golden State Warriors",
"LeBron James" : "Los Angeles Lakers",
"Kevin Durant" : "Brooklyn Nets",
"Giannis Antetokounmpo" : "Milwaukee Bucks",
}[val];
}
Objects are a powerful part of JavaScript, and they can help you simplify your code. Practice changing switch statements to objects and let me know how it goes! You can find me on: Twitter, LinkedIn, or Github